10 interesting stories served every morning and every evening.
The Federal Trade Commission today announced a final “click-to-cancel” rule that will require sellers to make it as easy for consumers to cancel their enrollment as it was to sign up. Most of the final rule’s provisions will go into effect 180 days after it is published in the Federal Register.
“Too often, businesses make people jump through endless hoops just to cancel a subscription,” said Commission Chair Lina M. Khan. “The FTC’s rule will end these tricks and traps, saving Americans time and money. Nobody should be stuck paying for a service they no longer want.”
The Commission’s updated rule will apply to almost all negative option programs in any media. The rule also will prohibit sellers from misrepresenting any material facts while using negative option marketing; require sellers to provide important information before obtaining consumers’ billing information and charging them; and require sellers to get consumers’ informed consent to the negative option features before charging them.
The final rule announced today is part of the FTC’s ongoing review of its 1973 Negative Option Rule, which the agency is modernizing to combat unfair or deceptive practices related to subscriptions, memberships, and other recurring-payment programs in an increasingly digital economy where it’s easier than ever for businesses to sign up consumers for their products and services.
Commission approval and publication follows the March 2023 announcement of a notice of proposed rulemaking which resulted in more than 16,000 comments from consumers and federal and state government agencies, consumer groups, and trade associations.
While negative option marketing programs can be convenient for sellers and consumers, the FTC receives thousands of complaints about negative option and recurring subscription practices each year. The number of complaints has been steadily increasing over the past five years and in 2024 the Commission received nearly 70 consumer complaints per day on average, up from 42 per day in 2021.
The final rule will provide a consistent legal framework by prohibiting sellers from:
* misrepresenting any material fact made while marketing goods or services with a negative option feature;
* failing to clearly and conspicuously disclose material terms prior to obtaining a consumer’s billing information in connection with a negative option feature;
* failing to obtain a consumer’s express informed consent to the negative option feature before charging the consumer; and
* failing to provide a simple mechanism to cancel the negative option feature and immediately halt charges.
Following an evaluation of public comments, the Commission has voted to adopt a final rule with certain changes, most notably dropping a requirement that sellers provide annual reminders to consumers of the negative option feature of their subscription, and dropping a prohibition on sellers telling consumers seeking to cancel their subscription about plan modifications or reasons to keep to their existing agreement without first asking if they want to hear about them.
The Commission vote approving publication of the final rule in the Federal Register was 3-2, with Commissioners Melissa Holyoak and Andrew N. Ferguson voting no. Commissioner Rebecca Kelly Slaughter issued a separate statement and Commissioner Holyoak issued a separate dissenting statement. Commissioner Ferguson’s dissenting statement is forthcoming.
FTC staff has developed a fact sheet summarizing the changes to the rule. The primary staffer on this matter is Katherine Johnson in the FTC’s Bureau of Consumer Protection.
...
Read the original on www.ftc.gov »
While Adobe’s annual MAX conference gives the company a chance to unveil its latest features, it also lets the brand demonstrate some of its more weird and wonderful ideas. ‘Sneaks’ sees Adobe engineers take to the stage to share ideas that may or may not see the light of day, such as 2024′s Project Turntable. Creative Bloq enjoyed an exclusive preview of the concept in ahead of its unveiling at MAX, and it’s one of the most impressive Sneaks we’ve seen.
Project Turntable lets users easily rotate 2D vector art in 3D, whilst ensuring it still look like 2D art from any new angle. And even after the rotation, the vector graphics stay true to the original shape, maintaining the design’s original essence.
In the example above, a 2D vector of an illustrated warrior is rotated to face a dragon. While spinning, the vector image appears to be a 3D object, but the static image the user settles on will be completely flat. Truly impressive is how the tool uses AI to fill in the ‘gaps’ in the image — in another example, a 2D horse with only two visibly legs is rotated to reveal four.
The tool was created by Adobe research scientist Zhiqin Chen. Adobe’s Brian Domingo told Creative Bloq that like other Adobe Innovation project, there’s still no guarantee that this feature will be released commercially — but the team expects it to generate a ton of interest at Adobe Max.
From Automatic Image Distraction Removal, and a new Generative Workspace, Adobe has already announced over 100 new creator-first features this week. And with huge announcements from other brands including Tesla and Meta, this has arguably been one of the biggest weeks for AI we’ve seen so far.
...
Read the original on www.creativebloq.com »
For the last seven years, I have been campaigning to establish and defend the public’s right to access all French national museums’ 3D scans of their collections, starting with the prestigious and influential Rodin Museum in Paris. My efforts have reached the Conseil d’État, France’s supreme court for administrative justice, and a great deal is at stake. The museum and the French Ministry of Culture appear determined to lie, break the law, defy court orders, and set fire to French freedom of information law in order to prevent the public from accessing 3D scans of cultural heritage.
Many museums around the world make high-quality 3D scans of important artwork and ancient artifacts in their collections. Several forward-thinking organizations freely share their 3D scans, allowing the public to view, copy, adapt, and experiment with the underlying works in ways that have never before been possible.
Anyone in the world with an internet connection can view, interact with, and download the British Museum’s 3D scan of the Rosetta Stone, for example. The public can freely access hundreds of scans of classical sculpture from the National Gallery of Denmark, and visitors to the Smithsonian’s website can view, navigate, and freely download thousands of high-quality scans of artifacts ranging from dinosaur fossils to the Apollo 11 space capsule.
With access to digitizations like these, artists can rework and incorporate our common cultural heritage into new works, such as films, video games, virtual reality, clothing, architecture, sculpture, and more. Researchers and educators can use 3D scans to further our understanding of the arts, and the art-loving public can use them to appreciate, study, and even replicate beloved works in new ways that are not possible within the confines of a museum or with the original works.
For example, I design and fabricate universal access wayfinding tools, interactive replicas and exhibits for the blind. I know that there is need for unfettered access to 3D scans of important sculptures and artifacts for use in making replicas the public can explore through touch.
If set loose to run free in our digital, visual, and tactile landscape, the creative potential for cultural heritage 3D scans is limitless, and the value of what the general public can do with them vastly exceeds what museums could ever create if they kept their digitizations for themselves.
Unfortunately, some ostensibly public-spirited organizations do keep their 3D scans hidden. I’ve been trying to help them see the light. Beginning in 2017 I spent three years using German freedom of information law to successfully pressure the Egyptian Museum of Berlin to release its 3D scan of its most prized possession and national treasure, the 3,000 year-old Bust of Nefertiti. Since then I’ve turned my attention to the digital treasures being hoarded by taxpayer funded institutions in France.
The Louvre, for example, will not allow the public to access its ultra-high quality 3D scan of Winged Victory, the Nike of Samothrace, despite its aggressive public and corporate fundraising campaign to digitize the iconic Greek sculpture. Nor its scan of Venus de Milo.
The French Ministry of Culture’s Réunion des musées nationaux (RMN) receives tens of millions of dollars anually in public subsidies to provide services to French national museums. In 2013 RMN received from the Fonds national pour la société numérique (FSN) a €1.1M subsidy and an additional loan of €1.1M to digitize objects in French museum collections and create a web platform for the publication and economic exploitation of its 3D scans. Since then RMN has 3D scanned thousands of artworks and ancient artifacts all over France. RMN advertises its scans’ availability to the public, which makes for great PR, but its ads are false. In fact, RMN has a strict look-but-don’t-touch policy for its 3D scans and absolutely refuses to allow the public to access them directly. My own investigation has revealed that, in private, RMN admits it won’t release its scans because it wants to protect its gift shops’ sales revenue from competition from the public making their own replicas. For practical applications and creative potential, and direct value to the public, it is as though these scans simply do not exist.
And then there is the Rodin Museum. Founded in 1917 shortly after the death of famed sculptor Auguste Rodin, le musée Rodin is a state-run administrative agency and an arm of the Ministry of Culture. It has a legally mandated mission to preserve, study, enhance and disseminate Rodin’s works, all of which have been in the public domain since their copyrights expired decades ago. Even though musée Rodin never passes up an opportunity to remind the public that it is France’s sole “self-funded” national museum, it sought and obtained direct public funding from the Ministry of Culture’s national digitization program, and in 2010 as part of its public service mission began 3D scanning its collection with the stated purpose of publishing the results.
Fourteen years later, musée Rodin’s scans have not been shared with the public.
When I approach cultural heritage organizations about their 3D scans of public domain works, my ultimate goal is to secure unfettered public access to them. I’m much less interested in half measures, whereby the public might be granted access to scans only for, say, educational purposes, but be improperly prohibited from using them commercially. Those kinds of compromises undermine the public’s right to reuse public domain works and can lead to people hesitating to use them in any way because they are unsure of their rights and fear unknown punishments.
In 2017, I approached musée Rodin with a strategy to elicit a full airing of every possible argument that a prestigious, well-positioned public institution might bring to bear against a member of the public interested in accessing its 3D scans. I wanted to discover and deal with all their concerns at once. So, from the start I made it perfectly clear to musée Rodin that I had a commercial interest in scans of Rodin’s works, and I made absolutely no apologies for it. From there I asked its administrators straightforward questions about the museum’s policies and let them treat me as they might treat any other inquiring member of the public. It did not go well.
I expected resistance, but I did not anticipate the extent to which the museum would abuse its authority.
After more than a year of musée Rodin’s administrators ignoring my requests for information about its 3D scans and public access-related policies, I decided to escalate. I asked for help from Paris-based civil rights attorney and strategic litigation expert Alexis Fitzjean Ó Cobhthaigh, who made a formal demand on my behalf under French freedom of information law, which requires government agencies to communicate their administrative documents to the public. We requested copies of the museum’s policy-related documents, a list of the 3D scan files it held, and the scan files themselves.
When musée Rodin refused to provide records of any kind we referred its refusal to the Commission on Access to Administrative Documents (CADA), the independent French government agency that administrations and courts rely on for its legal analysis of questions relating to public access and reuse of government documents. The CADA had never before considered any dispute about 3D scans. It affirmed my request that musée Rodin communicate copies of its scans to me, determining for the first time that public agencies’ 3D scans are in fact administrative documents and by law must be made available to the public.
In light of the government’s own legal analysis, musée Rodin confided to the ministry — in writing — its plan to disobey the law and its fear that I would bring it to court and make its position known to the public.
In 2019 we filed suit against the museum in the Administrative Tribunal of Paris, asking the court to anul the museum’s refusals and order it to make its scans public. Open culture and digital rights advocacy organizations Communia, La Quadrature du Net and Wikimédia France joined me as co-plaintiffs in support of our case. We were all represented by Fitzjean Ó Cobhthaigh.
After more than three years of litigation and musée Rodin’s desperate efforts to evade the law, in April 2023 the Administrative Tribunal of Paris issued a historic, precedent-setting decision, ordering the prestigious museum to make several of its 3D scans of some of the world’s most famous sculptures accessible to the public including The Thinker, The Kiss, and The Gates of Hell. The Administrative Tribunal’s April 21, 2023 decision is available here.
Our victory has broad implications for public access to and reuse of digitizations of important cultural heritage works throughout France and the world.
Naturally, we wanted to publicize the court’s 2023 decision at that time, but for strategic reasons remained silent because our victory was not complete. Despite the important rulings affirming the public’s right to access 3D scans, the tribunal also ruled against us on several related issues with reasoning that, if left unchallenged, threatens to broadly undermine French freedom of information law as well as permit the government to conceal a specific type of important document from the public — more on that in a moment.
When the tribunal issued its decision, Fitzjean Ó Cobhthaigh and I remained silent because we did not want to give the government advance notice that we intended to contest the flawed portions of the decision. We also wanted to quietly observe as musée Rodin’s deadline passed without it initiating an appeal of its own. In the months following the tribunal’s April 2023 decision, Fitzjean and I spent countless hours quietly researching, gathering expert testimony, and preparing an analysis and presentation of an appeal challenging the lower court’s legal and procedural errors. We engaged Fitzjean’s colleagues at the law firm SCP Marlange - de La Burgade — specialists in appeals to the Conseil d’État, France’s supreme court for administrative justice — for assistance and to present our case to the court. On December 1, 2023 we submitted our brief to the Conseil d’État for its preliminary review and its decision on the admissability of our appeal and the need for a hearing.
From the beginning of this seven-year effort, through today, musée Rodin has refused to give any comment to the press about its secret access policies or legal defense. In effect, the museum’s administrators have quietly transferred their press communications, policy decisions, and legal responsibilities to me, my attorney and co-plaintiffs, and the court, while making every effort to evade the law. But behind the scenes, in court, and when communicating with other arms of the government, however, musée Rodin has been relentlessly mendacious, lying to the Ministry of Culture, the government, the court, and the public it is meant to serve.
Now that we have challenged the lower court’s errors and await a response from the Ministry of Culture and its proxy, musée Rodin, I can finally give a full account of our important achievements in the tribunal and expose the museum’s deceptive tactics, all of which should be of interest to policymakers and professionals in the cultural heritage sector and the art market, open access advocates, as well as archivists, educators, and the art-loving public.
I can also now tell the public about the tribunal’s errors and lack of technical understanding and the danger they pose. What is currently at stake in my appeal to the Conseil d’État should be of particular interest to scientists, researchers, citizen activists, investigative journalists, and government watchdog organizations.
We had to fight the museum’s constant evasions, delays, deceptions, and lawless special pleading for each and every legal question we won. I recount below the three judges’ findings and their effects, some of musée Rodin’s failed legal and extralegal maneuvers, and how we countered them. I hope this summary will be helpful to others’ efforts to access documents of any kind from similarly uncooperative and hostile administrative agencies.
We definitively destroyed droit moral (moral rights) as a legal barrier to public access and reuse of 3D scans of public domain cultural heritage works. This is an incredible achievement in itself; no one in the world has a stronger claim to the legal protections and privileges of droit moral than musée Rodin, one of the most active, powerful, recognizable, and prestigious public domain artist’s estates in the world. The museum constantly reminded the court that it is the legal, state-appointed beneficiary of Auguste Rodin’s estate and perpetual moral rights, which include the right to attribution and the right to protect the integrity of an author’s works. For dramatic effect, musée Rodin submitted into evidence Rodin’s handwritten 1916 donation of his estate to the French state. Yet the court correctly considered droit moral to be utterly irrelevant to the public’s right to access and reuse works whose copyrights have expired and which have entered the public domain. This element of the court’s decision undercuts countless organizations’ hesitancy and dithering on public access to public domain works, sometimes vaguely justified as deference to artists’ estates and poorly understood, almost mystical claims of droit moral. Institutions like the Baltimore Museum of Art, for example, which has been too timid to publish its own 3D scan of The Thinker due to unfounded fear of somehow violating musée Rodin’s moral rights, should take note.
Musée Rodin argued that public access to its 3D scans would have “conséquences désastreuses” for all French national museums by subjecting their gift shop and artwork sales revenue to unfair competition from the public, and that their scans would facilitate criminal counterfeiting. The court ruled that the museum’s revenue, business model, and supposed threats from competition and counterfeiting are irrelevant to the public’s right to access its scans, a dramatic rejection of the museum’s position that makes the public’s right to access and reuse public domain works crystal clear. This element of the court’s ruling simultaneously neutralizes administrative agencies’ perpetual, self-serving pleas of poverty and denies their ability to withhold documents and cultural heritage digitizations based on prospective misuse by the public — including prospective criminal misuse by the person requesting access. On this point the court echoed the exact objection I made to musée Rodin’s then-director in my very first requests to the museum: courts, not administrative agencies nor museums, adjudicate crimes and only after a crime has been alleged, not in advance.
The court noted that musée Rodin had obviously created its 3D scans in the context of its public service mission to disseminate Rodin’s works and ruled the museum could not withhold them on the grounds that they would expose trade secrets related to their commercial operations. In an ironic development, the judges specifically reasoned against musée Rodin’s trade secrecy claim by citing its 3D digitization funding applications to the Ministry of Culture, in which the museum stipulated its commitment to publishing its scans. The museum had attempted to hide these funding applications from us and the court, telling the court they did not exist. However, in the course of the trial we obtained those applications by forcing a parallel documents request directly to the Ministry of Culture — which the museum complained to the court was a “crude maneuver” — exposing the museum’s deception and badly wounding the defense on this critical issue. Defeating the museum’s trade secrecy defense significantly narrows the scope of this legal exception to the public’s right to access 3D scans and other administrative documents, which other powerful cultural heritage organizations would likely attempt to abuse in similar cases.
The court rejected musée Rodin’s argument that it could not publish several 3D scan documents because they were in a closed format that required third-party software to open. By advancing this absurd defense, the museum revealed its utter hostility to public transparency, recklessly proposing a broad exception to the public’s right to access administrative documents that would render it completely useless. Imagine the damage to public oversight if the government could legally hide embarrassing documents merely by keeping them in file formats that required proprietary software to open. The court rejected the museum’s argument and specifically ordered it to make those same proprietary 3D files accessible to the public.
In the course of the trial, musée Rodin attempted to neutralize an element of our case by quietly publishing a small set of 3D scans on its website, including The Kiss and Sleep. It hoped that by publishing them it would remove them from the dispute and avoid the court ruling specifically on the communicability of 3D files in .STL format, commonly used in 3D printing. But in a strategic blunder, the museum could not resist editing these few files prior to publishing them. We alerted the court to these modifications, and it ruled not only that .STL files are communicable administrative documents, but also that the law requires the museum to communicate its original, unaltered scan documents.
Musée Rodin told the court that all of its other digital 3D scan files were “unfinished” — and therefore could lawfully be withheld from the public — because they had not been edited to include visible, indelible inscriptions of “Reproduction” on the sculptures’ scanned, digital surfaces. It claimed that the law against counterfeiting required such marks to prevent replicas from being misrepresented as originals and that without them, a digital, 3D scan document itself would constitute a criminal counterfeit. Those claims were nonsensical, of course, not only since it’s obvious that no one could mistake a digital file for a physical artifact, but also because vandalizing a scan by digitally carving the word “Reproduction” on it would be completely at odds with scanning practices in research, conservation, and cultural heritage contexts where fidelity to the original subject is paramount.
On the supposed legal requirement for “Reproduction” marks on 3D scans, the museum could only cite policy guidance from the Ministry of Culture, in the convenient form of a “Charter of best practices in 3D printing” produced by the ministry’s High Council of Literary and Artistic Property (CSPLA). Years earlier, when I had learned that the museum itself was contributing to the CSPLA’s policy recommendations, I alerted the CSPLA and objected to the museum’s obvious conflict of interest, warning that the museum would attempt to propose policies crafted specifically to retroactively oppose my requests for access. Later, in trial, as predicted, musée Rodin cited the CSPLA’s “charter” and submitted it as evidence in support of the idea that “Reproduction” marks are needed on 3D scans. The museum submitted this evidence without disclosing to the court that it had itself co-authored that self-serving — and, frankly, absurd — guidance.
The court completely rejected musée Rodin’s claims about marks of “Reproduction” on scans, echoing my earlier warnings to the CSPLA, specifically noting that the ministry’s policy recommendations were irrelevant because they had been issued years after the scans’ completion and could not be applied retroactively.
* Imperfection of scans and technological incompetence: defeated with evidence from London
Musée Rodin also argued that many of its 3D scan documents were incomplete and therefore non-communicable because they are in text format, have gaps in their surface measurements where the sculptures were not scanned in their entirety, and are generally mediocre in quality.
Incredibly, the museum also argued its scan documents were non-communicable because they are supposedly unusable since the museum’s own anonymous technical consultants claimed they did not know how to use them. An astonishing set of pleadings; imagine the thinking involved in arguing that documents may be concealed from the public because they are in a universally accessible text format, or because the administrative agency that holds them is too incompetent to use them.
In response to the museum’s nonsensical technological claims, we submitted expert testimony from Professor Michael Kazhdan, full professor of computer graphics in the department of computer science at Johns Hopkins University and co-developer of the Poisson Surface Reconstruction algorithm, which is used worldwide in 3D scan data analysis. Professor Kazhdan explained to the court that documents in plaintext format are fundamentally well suited for preserving scan data, and that such documents are easily exploitable by experts and amateurs alike. He also explained that gaps in scanned surfaces are a normal, expected, and ubiquitous phenomenon in 3D scanning and are not considered to render a scan document “incomplete” or unusable.
We also submitted testimony from artist and 3D scanning expert Ghislain Moret de Rocheprise, who explained that plaintext format is the exact format the Ministry of Culture itself recommends for 3D scanned point-cloud documents and that it is commonly used by the general public as well as industry and government precisely because it is open, non-proprietary, and easy to preserve and access.
On the question of the supposed uselessness of so-called incomplete 3D scans, we also submitted as evidence correspondence from 2020 between musée Rodin and the Tate London museum, which I obtained through a separate UK Freedom of Information Act request to the Tate. In that correspondence, Tate requested permission to 3D scan several sculptures on loan from musée Rodin, so that Tate could create digital renders of the works for use in a promotional video. Musée Rodin granted Tate that permission on the express condition that the sculptures not be scanned in their entirety. This directly contradicted musée Rodin’s position in court, demonstrating that it fully recognizes the utility of so-called “incomplete” scans. Musée Rodin’s preferential treatment of Tate London also revealed that it well understands there is no legal requirement to append marks of “Reproduction” to 3D scans of Rodin’s works.
Evaluating our evidence and the museum’s strange claims, the court determined that musée Rodin cannot evade its obligation to communicate 3D scans by claiming they exclude portions of the scanned subjects or are of low quality, nor by admitting its technological incompetence to use them.
The court viewed musée Rodin’s trademarks in the “Rodin” name to be irrelevant, despite the museum’s repeated pleadings. This decision will help prevent other organizations from blocking public access to artwork by attaching their trademarks to digital records and physical replicas of public domain works.
Musée Rodin had originally assured the CADA that it would comply with my request for the museum’s internal correspondence discussing my first requests to the museum. Once in trial, though, the museum reversed its position and attempted to conceal these documents from me with an improper, retroactive claim of attorney-client privilege which the judges rejected. The court ordered musée Rodin to communicate to me its early, internal deliberations on my initial inquiries.
Before I filed my suit, in correspondence between musée Rodin and the Ministry of Culture — which the museum accidentally revealed during trial — the museum’s then-director asked the Ministry for advice on how the museum should respond to my requests and defend its refusals. The Ministry declined to give the museum any guidance, noting the Ministry’s own need for the clarifications from the court that would result from my likely litigation. Despite this, and even though musée Rodin reluctantly made several concessions to me only in the course of the trial and acknowledged it was doing so as a direct result of my suit, the museum nonetheless petitioned the court to condemn me to pay for its legal defense, and requested a figure more than ten times what is typical in this kind of procedure. This maneuver was clearly intended to penalize and intimidate me and discourage future requesters from standing up for the public’s rights.
Not only did the court reject that abusive request, the judges condemned musée Rodin to pay me € 1,500 towards the expenses I incurred in bringing this action against the museum. Even though this a very small, symbolic sum compared to the time and effort my attorney and I have devoted to this effort, we are happy to have this recognition from the court of the legitimacy and need for our efforts.
As of this writing, more than a year past the court’s deadline to communicate its scans and related documents to the public, musée Rodin has still not complied; it has not published nor sent me a single document, nor has it paid me the token compensation. The museum has apparently decided to compound its lawlessness by defying the court’s orders, likely with the intent to exhaust our very limited resources by forcing us to bring it back to the tribunal to get another order forcing it to actually comply with the ruling.
Nevertheless, altogether the court’s decision amounts to a complete repudiation of all arguments against public access that relied on the museum’s droit moral, economic model, cultural and patrimony sensitivities, institutional prestige and romantic mystique. We accomplished this despite the museum’s false alarms of an existential threat to all French national museums, and we did this with a foreign plaintiff (me), whom musée Rodin portrayed as a direct commercial competitor, and whom it repeatedly insinuated had criminal intent, a notion we did not even credit with a denial. Our entire approach and framing of the case, and the resulting decision, clear away the government’s strongest, most sensational — and most ludicrous — objections to public access to digitizations of public domain artwork.
We have established the strongest legal foundation for public access and reuse of digitizations of public domain works ever, in the most challenging possible circumstances, against an extremely well-positioned adversary. This ruling is a significant accomplishment that will help with every other fight for public access to cultural heritage works in France and beyond.
Along with the important precedents described above, however, the tribunal made several serious legal and procedural errors that Fitzjean Ó Cobhthaigh and I feel obligated to correct. If those errors stand, they would weaken the public’s right to access administrative documents in general, and specifically permit the government to conceal an extraordinarily important class of documents. The tribunal’s errors pose a danger to the public well beyond the question of accessing simple 3D scans from museum collections.
From the very start, I had requested that musée Rodin communicate to me a list of the 3D scan files in its possession, including scans of works from other organizations’ collections. The CADA’s analysis affirmed my request, noting that the law requires the museum to communicate such a list to the public even if one does not exist and must be generated on demand. In response, the museum not only denied that it held scans from other organizations, but it flatly told the CADA — in writing but without any justification — that it simply would not give me a list of any scan files it held. In court it never offered any defense of this lawless position.
Instead, during trial musée Rodin provided two incomplete, imprecise, and contradictory inventories, not of files, but of the few sculptures it admits having scanned and partial, vague descriptions of their file formats. At the same time, the museum complained to the court that our request for documents lacked sufficient precision since we could not guess at the exact files it held.
We repeatedly urged the court to recognize that the museum had in fact never supplied a simple list of files and had never identified even a single scan file by its actual file name.
The court nonetheless ruled that my request for a list of files had been rendered moot because the museum had provided one during the trial, which, again, it quite simply never did.
To this day, the court itself has no idea what scan files the museum possesses nor what, specifically, it has ordered the museum to publish, and the public can only guess. It is a very strange situation.
In its first defense brief, musée Rodin confirmed that it held 3D scans of several sculptures, including The Kiss, Sleep, and The Three Shades. It specifically told the court it would publish these three scans in order to neutralize elements of my suit. While it eventually, improperly, published edited versions of The Kiss and Sleep, it published nothing for The Three Shades.
We not only alerted the court to musée Rodin’s failure to publish the The Three Shades scan, but we were also able to locate and present the court with photographic evidence that the museum had indeed scanned the sculpture.
The museum responded in its second defense brief by reluctantly elaborating that in 2008 it had cooperated with several organizations to 3D scan a plaster cast of The Three Shades that belongs to the National Foundation for Contemporary Art (FNAC) and has been deposited in the musée des Beaux-Arts de Quimper since 1914, before Auguste Rodin died and before musée Rodin was even established. Musée Rodin explained to the court that it had mistakenly promised to publish a scan of The Three Shades only to realize later that it did not have a scan of its own cast of the sculpture. Obviously worried that it had accidentally confirmed that it holds scans from other organizations’ collections after specifically denying this, the museum further explained… nothing.
Musée Rodin never suggested there had been any technical problems when it scanned FNAC’s Three Shades. It never suggested it could not locate the scan. It never claimed the scan did not exist, and it never denied possessing it. The Three Shades simply disappeared from musée Rodin’s defense arguments, and for some reason the court took it upon itself to imagine an explanation for its vanishing.
Incredibly, in its written decision, the tribunal ruled that my request for the scan of The Three Shades was moot because, in the judges’ own words, “musée Rodin ultimately maintains that its research shows that it did not proceed with the digitalization of The Three Shades due to technical difficulties. Under these conditions, no files can be communicated.”
It’s fitting that The Three Shades stands atop the Gates of Hell. The court’s mishandling of a simple list of files and The Three Shades together would damn the public’s right to access administrative documents to a hopeless fate. If on one hand the court may credit an administrative agency with identifying files it never identified, while on the other hand erroneously conclude that other files never existed — without the agency even making that claim and despite the agency’s own statements and independent proof to the contrary — the government could conceal any documents it pleased simply by being strategically sloppy with its statements to the court, rendering freedom of information law completely dysfunctional.
The potential for harm from these errors is difficult to overstate, since it is multiplied by the court’s further confusion over a simple type of document commonly used to record and preserve 3D measurements: point-clouds. At a minimum, the documents and data at risk relate to the entirety of the French national territory.
As we carefully explained to the tribunal, when a subject is 3D scanned the scanning equipment takes measurements of many millions — sometimes billions — of points on the subject’s surface. A document known as a point-cloud is saved, which records the X, Y, and Z positions of each measured point relative to the scanner. Sometimes they also record each point’s color data and other properties. Point-cloud documents are the primary, fundamental metrological records that archive the results of 3D scan surveys. They are the essential documents from which all analyses and understanding flow and are typically among the most important deliverables scanning service providers produce for their clients, be they private or public.
Because they are so important, point-clouds are frequently saved in plaintext formats like .ASCII, .OBJ, and .TXT to ensure that they remain open source, easily accessible, and well-preserved for future generations. Anyone can open a plaintext formatted point-cloud document in text editing software and view the numeric values it records. Point-cloud documents are of course directly exploitable with 3D software and simple web-browser visualization tools which can easily and immediately read them and display visualizations, 3D digital environments and reconstructions, and precise analyses of the scanned subject. The Ministry of Culture itself recommends preserving and publishing scan data in open text format point-clouds for these very reasons, and this is also why musée Rodin originally agreed to create and publish text-formatted point-clouds in its digitization funding applications to the ministry so many years ago.
So what is really at risk here?
Our case deals with scans of sculptures held by musée Rodin — perhaps a few dozen, perhaps hundreds; the number is unclear — but the lower court did not seem to realize that 3D scanning isn’t limited to sculpture or the cultural heritage sector, of course. Point-cloud documents are used to record and preserve information about almost every type of subject worth scanning, across countless important sectors of intense public interest.
France’s National Institute of Geographic and Forestry Information (IGN), for example, is undertaking an ambitious aerial 3D scanning survey of “the entire soil and subsoil of France.” Forests, farmland, coastlines, roads, civil infrastructure and entire cities — the entire built and natural environment — are being 3D scanned and recorded in point-cloud documents.
According to IGN, trillions of points of 3D data are being gathered “to respond to major societal challenges” and all the data being acquired and produced within the framework of the program is being distributed under open data licenses. IGN’s point-cloud documents are intended to be used in environmental monitoring, risk management, forestry and agriculture, biodiversity monitoring, municipal and infrastructure planning, archaeology, and whatever else the public wishes to do with them, including uses we have not yet invented.
Point-cloud documents are also used in specific cases we cannot anticipate, including assessing disaster sites, crime scenes, and industrial accidents. For example, point-clouds of Notre Dame de Paris resulting from 3D surveys made prior to the 2019 fire are now essential to its current restoration.
In our case, the administrative tribunal of Paris appears to have disregarded these incontrovertible facts and our experts’ advisories on the role, usefulness, universal accessibility, direct exploitability, and importance of point-cloud documents. It also ignored our request that it undertake an investigation of its own to better inform itself and understand the technology and issues at stake.
Instead, the court appears to have allowed itself to be confused by the unsubstantiated and contradictory statements of musée Rodin’s anonymous and confessed incompetent technical consultants.
The court articulated its profound misunderstanding of the technology and nature of point-cloud documents by explaining that they may be withheld from the public because they are not directly useful in themselves, “but only constitute raw material needed for the production of subsequent documents.”
The court not only deferred to the museum’s misleading technical characterizations, it did something far more strange and ominous. On its own initiative the tribunal ruled that since it contains unedited raw data, a document containing a point-cloud — an entire class of documents — may be withheld from the public because, as the court explained, it “does not reveal any intention on the part of the administration”.
Worse, during trial, musée Rodin itself did not even suggest either of these strange technical and legal arguments, which only appeared for the first time in the judges’ own final written decision. My attorney and I were never afforded an opportunity to point out to the court that its reasoning had no basis in the law, which holds that an administration’s “intentions” are irrelevant to the communicability of its documents. Nor could we gently remind the court that its factual assertions were untrue and wildly at odds with 3D scanning and 3D data handling practices and consensus in the cultural heritage field, in industry, by amateurs, and with the government’s own recommendations for 3D data preservation and dissemination.
The tribunal’s legal and technological errors on the point-cloud issue alone pose a threat to public access to millions of documents containing petabytes of data from government-funded 3D surveys of the environment, oceans, the atmosphere, forests, floodplains, farmland, architecture, towns and cities, civil infrastructure, and archaeological sites, as well as the primary, archival, highest-resolution 3D scans of cultural heritage works.
Under the tribunal’s ruling, publicly funded point-cloud documents such as those being produced by IGN and others could legally be withheld entirely from the public. Or, the government could elect, at its own discretion, to grant the public access to such documents only after they had been processed, refined, or edited to accommodate private contractors’ and special interests’ commercial considerations, or perhaps political sensitivities, with no legal recourse for the public to examine the original, unaltered point-cloud documents directly.
Concerning point-clouds alone, the court’s errors immediately threaten to stall important technological developments, hinder scientific research and openness, and obscure and impede infrastructure and environmental monitoring by scientists, the public, journalists, and the general public.
I can only imagine and hope that the tribunal was simply unaware of the scope and degree of damage these highly irregular elements of its decision would cause. They are dangerous.
France’s supreme court formally accepted our appeal on April 17, 2024 and transmitted our brief to musée Rodin and the Ministry of Culture. You can read our brief here. The court gave the ministry and museum two months to present their defense, a deadline they have, as of this writing, missed by over three months. It is unlikely they will publicize their defense nor solicit input from the public while drafting it.
Our brief explains point-by-point the Paris tribunal’s grave legal and procedural errors and unambiguously demonstrates the lower court’s obvious and profound technological misunderstanding of point-cloud documents.
So much more is now at stake than public access to 3D scans of a few popular sculptures, or preserving the 19th-century business model of one intransigent museum. One museum could have lawless and reactionary administrators opposed to public oversight and public access, with no vision or planning for the future or innovation in the arts, and the damage might be reasonably contained to the loss of scans of its own collection. But with its deceptive arguments, reckless tactics, and the approval of the Ministry of Culture, musée Rodin managed to distract and confuse the tribunal with dangerous implications on a much larger scale.
The public and the Conseil d’État need to understand that the tribunal’s reasoning would fundamentally undermine the public’s right to access administrative documents and hinder its ability to monitor the government’s activities in countless sectors. The lower court’s errors would subject the public’s right to access administrative documents to the arbitrary whims of administrations that could simply hide, obfuscate, or misrepresent their holdings and their “intentions” for documents as a means of blocking public inquiry. The tribunal’s decision would also set a precedent allowing regional courts to simply invent their own legal standards on a case-by-case basis, based on total unfamiliarity with both well-established and emerging technologies, without giving litigants or the public a chance to respond.
All this public damage because musée Rodin wants to operate like a private atelier with a monopoly to exclusively service one long-dead artist’s estate and his wealthy collectors, forever. Rather than embrace the future and permit the public to access their 3D digitizations of the public’s cultural heritage, musée Rodin and the Ministry of Culture have created a smokescreen of legal and technological nonsense and started a fire that threatens our common resources across the entire digital landscape.
The productive, lawful elements of the Paris tribunal’s decision will be essential to securing access to countless important 3D scans from French national museums. The decision will help others’ efforts to modernize policies and establish public access to digitizations in public institutions throughout Europe. We can all look forward to what the public will create by fully exercising our newly clarified rights, and we will all be enriched by the full use of 3D scans of our shared cultural heritage.
But first the Conseil d’État must extinguish the fire musée Rodin and the Ministry of Culture have started.
Cosmo Wenman is an open access activist and a design and fabrication consultant. He lives in San Diego. He can be reached at cosmowenman.com and twitter.com/CosmoWenman
If you enjoyed this story, please share it and subscribe. I’ll be writing more about this case as it progresses.
Hélène Pilidjian
Head of the litigation office,
Ministry of Culture
helene.pilidjian@culture.gouv.fr
+33 (0)1 40 15 80 00
Decision of the Administrative Tribunal of Paris, April 21 2023:
...
Read the original on cosmowenman.substack.com »
Web browsers are ubiquitous, but how do they work? This book explains, building a basic but complete web browser, from networking to JavaScript, in a couple thousand lines of Python.
Web Browser Engineering will be published by Oxford University Press before the end of the year. To get it as soon as it’s out, pre-order
now!
Follow this book’s blog or Twitter for updates. You can also talk about the book with others in our discussion
forum.
If you are enjoying the book, consider supporting us on Patreon.
Or just send us an
email!
...
Read the original on browser.engineering »
This site is home to the documentation for the SQLite project’s WebAssembly- and JavaScript-related APIs, which enable the use of
sqlite3 in modern WASM-capable browsers.
Cookie/storage disclaimer: this site requires a modern, JavaScript-capable browser for full functionality. This site uses client-side storage for persisting certain browsing preferences (like the bright/dark mode toggle) but its server does not process, nor transfer to any other entity, any user-level information beyond certain SCM-side display-related preferences and the credentials of logged-in developers.
Making use of this project:
Third-party projects known to be using this project include (in order of their addition to this list)…
* Evolu Evolu is a local-first platform
designed for privacy, ease of use, and no vendor lock-in.
* SQLiteNext provides a demo of
integrating this project with next.js.
* sqlite-wasm-esm demonstrates
how to use this project with the Vite toolchain.
* sqlite-wasm-http
provides an SQLite VFS with read-only access to databases which are
served directly over HTTP.
The following links reference articles and documentation published about SQLite WASM by third parties:
...
Read the original on sqlite.org »
<center>
<img src=“https://docs.monadical.com/uploads/213b6618-e133-4b6b-a74a-267de68606aa.png” style=“width: 140px”>
# Big changes are coming to ArchiveBox!
*New features coming to the future of self-hosting internet archives: a full plugin ecosystem, P2P sharing between instances, Cloudflare/CAPTCHA solving, auto-logins, and more…*.
</center>
<hr/>
In the wake of the [recent attack](https://www.theverge.com/2024/10/9/24266419/internet-archive-ddos-attack-pop-up-message) against Archive.org, [ArchiveBox](https://archivebox.io) has been getting some increased attention from people wondering how to **self-host their own internet archives**.
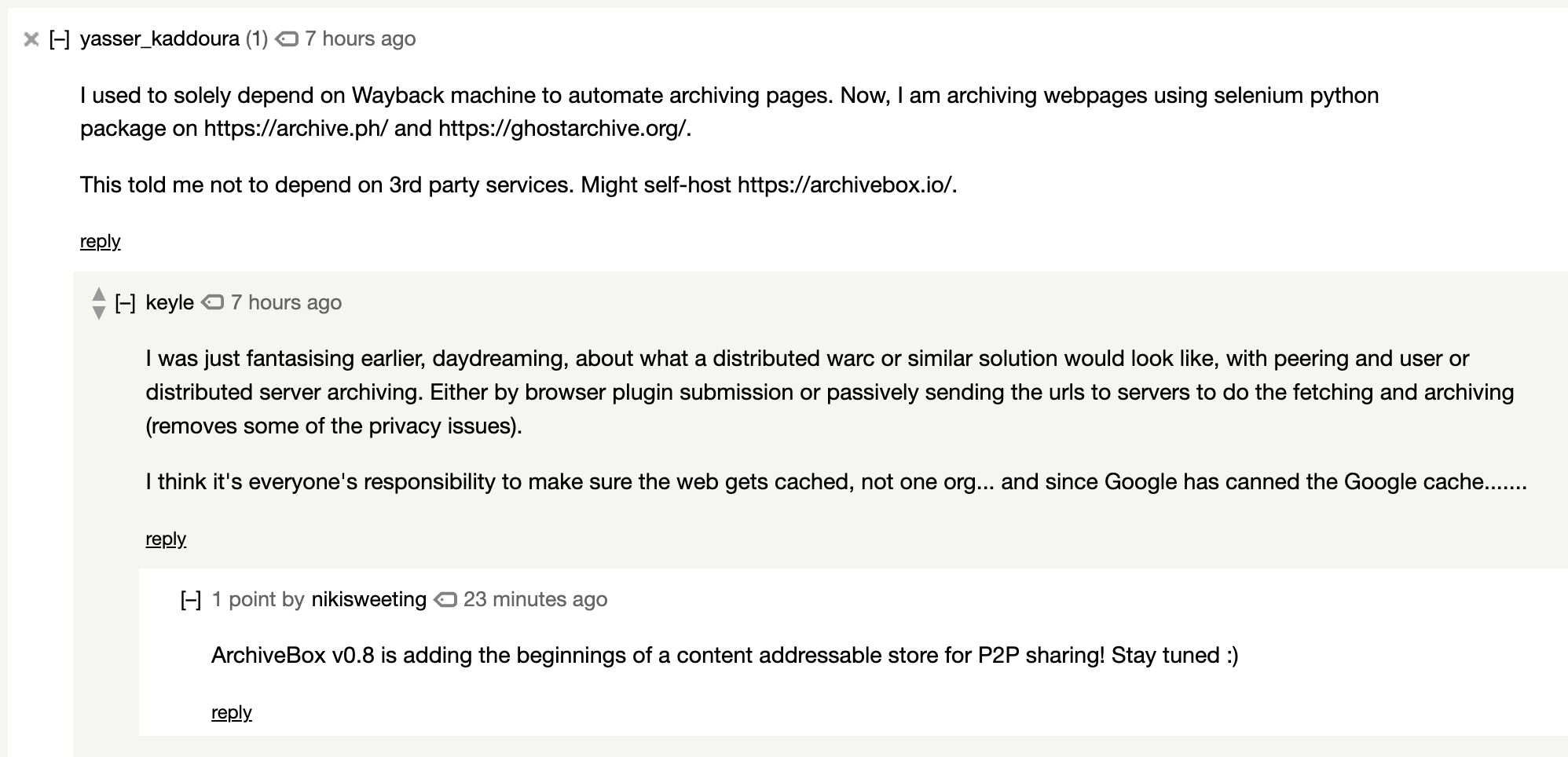
ArchiveBox is a strong supporter of Archive.org and their mission to preserve all of human knowledge. We’ve been donating for years, and we urge you to do the same, they provide an invaluable service for all of humanity.
We completely condemn the DDoS and defacement of their site, and hope it never happens again. Realistically though, they are an attractive target for people who want to supress information or [start IP lawsuits](https://blog.archive.org/2024/07/01/what-happened-last-friday-in-hachette-v-internet-archive/), and this may not be the last time this happens…
<br/>

<br/>
> We envision a future where the world has both a robust centralized archive through Archive.org, and a widespread network of decentralized ArchiveBox.io instances.
<center>
<a href=“https://github.com/ArchiveBox/ArchiveBox”><img src=“https://docs.monadical.com/uploads/bac22f63-0fc9-4634-8fb8-67d29806e49c.png” style=“max-width: 380px; border: 4px #915656 solid; border-radius: 15px; box-shadow: 4px 4px 4px rgba(0,0,0,0.09)“/></a>
</center>
<br/>

<br/>
### The Limits of Public Archives
In an era where fear of public scrutiny is very tangible, people are afraid of archiving things for eternity. As a result, people choose not to archive at all, effectively erasing that history forever.
We think people should have the power to archive what *matters to them*, on an individual basis. We also think people should be able to *share* these archives with only the people they want.
The modern web is a different beast than it was in the 90′s and people don’t necessarily want everything to be public anymore. Internet archiving tooling should keep up with the times and provide solutions to archive private and semi-private content in this changing landscape.
#### Who cares about saving stuff?
All of us have content that we care about, that we want to see preserved, but privately:
- families might want to preserve their photo albums off Facebook, Flickr, Instagram
- individuals might want to save their bookmarks, social feeds, or chats from Signal/Discord
- companies might want to save their internal documents, old sites, competitor analyses, etc.
<sub>*Archiving private content like this [has some inherent security challenges](https://news.ycombinator.com/item?id=41861455), and should be done with care.<br/>(e.g. how do you prevent the cookies used to access the content from being leaked in the archive snapshots?)*</sub>
#### What if the content is evil?
There is also content that unfairly benefits from the existence of free public archives like Archive.org, because they act as a mirror/amplifier when original sites get taken down.
There is value in preserving racism, violence, and hate speech for litigation and historical record, but is there a way we can do it without effectively providing free *public* hosting for it?
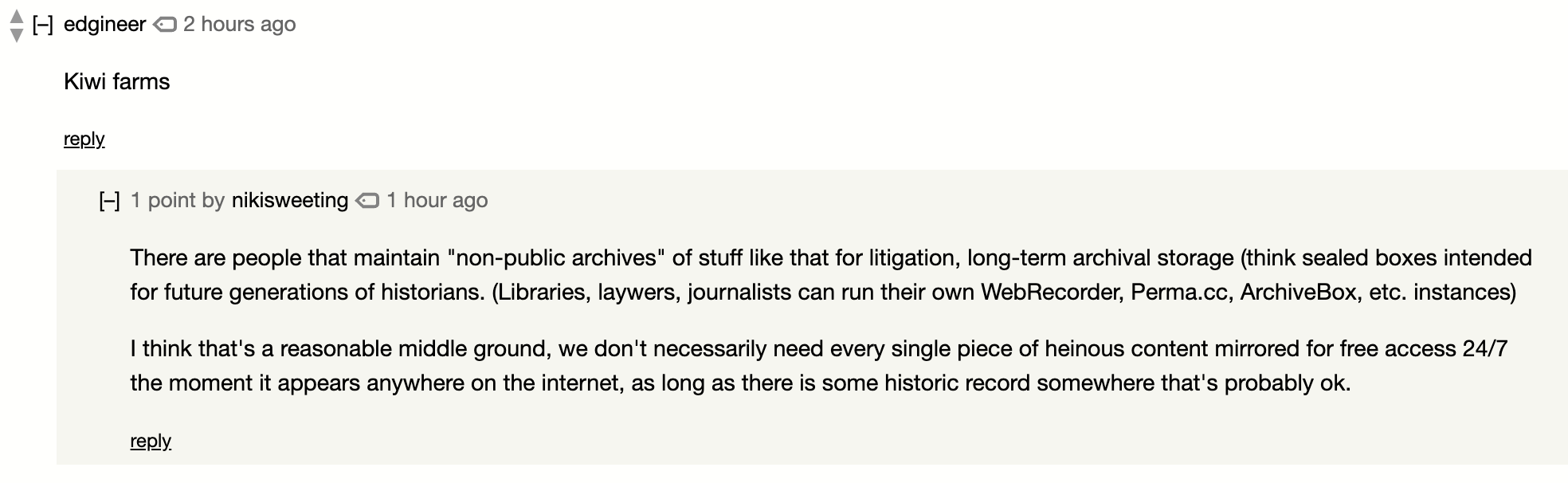
<br/>
<center>
## ✨ Introducing ArchiveBox’s New Plugin Ecosystem ✨
</center>
<br/>
[ArchiveBox v0.8](https://github.com/ArchiveBox/ArchiveBox/releases) is shaping up to be the [**biggest release in the project’s history**](https://github.com/ArchiveBox/ArchiveBox/pull/1311). We’ve completely re-architected the internals for speed and performance, and we’ve opened up access to allow for a new plugin ecosystem to provide community-supported features.

We want to follow in the footsteps of great projects like [NextCloud](https://apps.nextcloud.com/) and [Home Assistant](https://www.home-assistant.io/addons/), and provide a robust “app store” for functionality around bookmark management, web scraping, capture, and sharing.
#### 🧩 Here’s just a taste of some of the first plugins that will be provided:
- `yt-dlp` for video, audio, subtitles, from Youtube, Soundcloud, YouKu, and more…
- `papers-dl` for automatic download of scientific paper PDFs when DOI numbers are seen
- `gallery-dl` to download photo galleries from Flickr, Instagram, and more
- `forum-dl` for download of older forums and deeply nested comment threads
- `readability` for article text extraction to .txt, .md, .epub
- **`ai`** to send page screenshot + text w/ a custom prompt to an LLM + save the response
- **`webhooks`** trigger any external API, ping Slack, N8N, etc. whenever some results are saved
- and [many more…](https://github.com/ArchiveBox/ArchiveBox/tree/dev/archivebox/plugins_extractor)
If you’re curious, the plugin system is based on the excellent, well-established libraries [pluggy](https://pluggy.readthedocs.io/en/stable/index.html) and [pydantic](https://pydantic-docs.helpmanual.io/). It was a fun challenge to develop a plugin system without over-engineering it, and it took a few iterations to get right!
> I’m excited for the future this will bring! It will allow us to keep the **core** lean and high-quality while getting community help supporting a **wide periphery** of plugins.
<br/>
#### ✨ Other things in the works:
- There is an all-new [`REST API`](https://demo.archivebox.io/api) built with `django-ninja`, already [available in BETA](https://github.com/ArchiveBox/ArchiveBox/releases)
- [Support for external storage](https://github.com/ArchiveBox/ArchiveBox/wiki/Setting-Up-Storage) (AWS/B2/GCP/Google Drive/etc.) (via `rclone`) was added
- We’ve started adding the beginnings of a content-addressable store system with unique “ABID”s (identifiers based on URL + timestamp) that can be shared between instances. This will help us build BitTorrent/IPFS-backed P2P sharing between instances in the future.
- We’ve added a background job system using [`huey`](https://huey.readthedocs.io/)
- new auto-install system `archivebox install` (no more complex `apt` dependencies)
*(plugin’s cross-platform runtime dependencies are very hard to package and maintain, check out our new [`pydantic-pkgr`](https://github.com/ArchiveBox/pydantic-pkgr) library that solves this and use it in your projects!)*
> ArchiveBox is designed to be local-first with [**SQLite**](https://www.sqlite.org/famous.html), P2P will always be optional.
<br/>
#### 🔢 For the minimalists who just want something simple:
If you’re an existing ArchiveBox user and feel like this is more than you need, don’t worry, we’re also releasing a new tool called [`abx-dl`](https://github.com/ArchiveBox/abx-dl) that will work like like `yt-dlp` or `gallery-dl`.
It will provide a one-shot CLI to quickly download *all* the content on any URL you provide it without having to worry about complex configuration, plugins, setting up a collection, etc.
<br/>
<br/>
### 🚀 Try out the new BETA now!
```bash
pip install archivebox==0.8.5rc44
archivebox install
# or
docker pull archivebox/archivebox:dev
📖 *Read the release notes for the new BETAs on our [Releases](https://github.com/ArchiveBox/ArchiveBox/releases) page on Github.*
💬 *[Join the discussion on HN](https://news.ycombinator.com/item?id=41860909) or over on our [Zulip forum](https://zulip.archivebox.io/).*
💁♂️ *Or [hire us](https://github.com/ArchiveBox/archivebox#-professional-integration) to provide digital preservation for your org (we provide CAPTCHA/Cloudflare bypass, popup/ad hiding, on-prem/cloud, SAML/SSO integration, audit logging, and more).*
<br/><br/>
<img src=“https://docs.monadical.com/uploads/a790d511-db5c-49ae-a3d9-db41e4100f20.png” style=“width: 19.9%“/><img src=“https://docs.monadical.com/uploads/4233a584-3903-4610-9016-06d1b59ac682.png” style=“width: 19.9%“/><img src=“https://docs.monadical.com/uploads/63a73bfc-f028-40c4-885c-ad011c7e191a.png” style=“width: 19.9%“/><img src=“https://docs.monadical.com/uploads/e621d80f-87d4-4ade-8fb9-9e0e6ab42a7f.png” style=“width: 19.9%“/><img src=“https://docs.monadical.com/uploads/26285bd6-f240-41e3-99b7-70d50463e3b7.png” style=“width: 19.9%“/>
<center>
[Donate to ArchiveBox](https://hcb.hackclub.com/donations/start/archivebox) <sup>(tax-deductible!)</sup> to support our open-source development.<br/><br/>Remember to also donate to [Archive.org](https://help.archive.org/help/how-do-i-donate-to-the-internet-archive/) <sup>(not affiliated)</sup> to help them with the attack!
</center>
...
Read the original on docs.sweeting.me »
Skip to main content
What you’re seeing below is two of Crokinole’s greats simultaneously securing perfect rounds. Technically speaking, they each flicked a 3.2cm disc 30cm across a board into a 3.5cm hole (just 9% wider than the disc itself) eight times in a row. In game terms, they made eight open 20s each. But it’s just flicking a little disc across a small board. How hard can it be, really? The mesmerizing 56 seconds above were captured at the semifinals of the 2024 World Crokinole Championship, where Connor Reinman defeated Justin Slater. A matchup not unlike Magic vs. Bird, or Swift vs. Eilish. How rare was this feat of perfection? Was this one of those obscure new Olympic events? You may even be wondering, wtf is Crokinole? We’ll get to all these questions. But first, you must understand Crokinole. The game’s origin: the southern region of Ontario. If you are from the southern region of the Canadian province of Ontario, you may already be well-versed in Crokinole due to its Canadian origin. For the uninitiated, Crokinole is like a mashup of shuffleboard and curling, played on a tabletop board. It’s been around since the 19th century but has seen a steady rise in global popularity in recent years. To know the game, one must play the game.
Let your training begin. The main objective is to flick your discs into higher-scoring regions. The center hole is worth 20 points. Be careful, though—eight pegs surround the fifteen-point region. Here, your opponent shoots on an empty board. To be a valid shot (and stay on the board) the disc must land within the 15-point region. If any opponent discs are on the board, your disc must hit one to be valid and stay on the board. Give it a try: Hit your opponent’s disc. Use the slider and button below the board to position your disc. Then aim, hold the shoot button to find the perfect power, and release. If you shoot and it is an invalid shot, your disc is removed from the board and is not scored. It’s your turn, and there are no opponent discs. You just need to land in the fifteen-point region, but scoring a 20 is ideal. A made 20 is set aside and counted. Give it a try: Shoot your disc into the center hole to secure the 20 points. After all sixteen discs are played, points are tallied based on the regions and any 20s. The round winner is the player with the most points, and a game consists of multiple rounds. Easy Keanu, that was just the basics. We didn’t even get to the one-cheek rule (yes, that cheek). For more details you can watch this video or read the rules. Oh, and feel free to go play—we made a simulator for you to hone your skills against a bot. You are ready for the next part of the journey. What does the data tell us about Connor Reinman and Justin Slater? Reinman, the back-to-back world champion, and Slater, the perennial powerhouse, are arguably the greatest players right now on the world stage. Player rankings according to Crokinole Reference. No matches from 2021-2022. But how good are they? Let’s start by looking at their ability to make open 20s, an indispensable skill for success. Here’s how competitive players compare in open 20 success rates. Reinman and Slater are top competitors in open 20s, with success rates of 66% and 75%, compared to the average competitive player’s 55%. For basketball fans: a Crokinole player making eight consecutive 20s in live play is like an NBA player sinking 20 straight free throws during a game—not impossible, but far from common. How do they compare to casual players? Observing players with varying experience, success rates for in-game open 20s ranged from 20% to 50%. The odds of two opponents making eight consecutive shots can vary greatly depending on their skill level. Here are the odds of a double perfect round. Our theoretical scenarios show how even a slight drop in skill greatly impacts the odds. To witness this rare event, both top players must hit a hot streak at the same time. These percentages reflect in-game attempts, where a player’s rhythm is disrupted by various shots. In non-competitive, less plamigerent settings, their skills really shine—like Shawn Hagarty, who set an unofficial record with 64 consecutive open 20s. However, real games are far more nuanced and complex. Players — or their opponents — often miss early on. Here’s what the data reveals after analyzing 300 rounds from various matchups in last season’s tournaments. At Which Shot an Open 20 is First Missed in Competitive Matches Note: Based on 300 rounds from final to quarterfinal matches in the past year. More often than not, the elusive double perfect round is lost right at the start. But I’ve been discussing this in the context of the most elegant form — a “pure” double perfect round, where all 16 shots are made as open 20s. Technically, though, a miss doesn’t completely rule out a perfect round. A perfect round can (and often does) include a combination of open 20s and ricochet 20s, where a disc bounces in off the opponent’s. Ricochet 20s by Justin Slater and Andrew Hutchinson. Watch on YouTube. The perfect blend of aim and power is required to perfect shots like those. Try it yourself: can you achieve the feel-good ricochet 20? Going for a 20 isn’t always the best or even a viable option. Discs on the board introduce more exciting scenarios that add layers of strategy. Having all your discs on your side is usually a strong defensive position, but watching your opponent dismantle it can be demoralizing. That’s exactly what happened in this round between Andrew Hutchinson and Nolan Tracey. The Slater-Reinman round was the only double perfect in a review of 445 highly competitive rounds in the past year. One thing is certain: more skilled players tend to keep the board clear and make open 20s at a higher rate, increasing the chance of glimpsing a pure double perfect round. If there’s one takeaway, it’s that Crokinole is fun and the community is awesome. Whether you’re playing to win or just flicking a few rounds with Grandma during the holidays, it’s a great time. So, maybe you’re into Crokinole now? Here are some resources to help you get started, or go get some practice in with the simulator. Buy a board here (or here, here, here) Player types are estimations based on all open 20 success rates from 2023-2024 NCA tournament data. Competitive 20 success rates are from Shawn Hagarty’s impressive data. Recreational open 20 success rates are based on observations of 600 open 20 attempts from 10 individuals with at least 50 attempts each. First missed shot data is from watching playoff-rounds from all 2023-2024 tournaments on Tracey Boards coverage of events. Yearly NCA tour rankings data is based on the rank in July (at the end of the season). Data from Crokinole Reference. The Pudding
is a digital publication that explains ideas debated in culture with visual essays.
...
Read the original on pudding.cool »
AI generated, not affiliated with Hacker News or Y Combinator.
...
Read the original on hnup.date »
The new Kindle Scribe offers a first-of-its-kind in-book writing experience and a more powerful notetaking experience. With Active Canvas, you can write your thoughts directly in the book when inspiration strikes. Your note becomes part of the page, and the book text dynamically flows around it—if you increase the font size, change the font style, or the book layout changes, the note remains visible exactly where you want it so you never lose any meaning or context. Coming soon, you’ll also be able to write your notes in the side panel and easily hide them when you are done. The all-new, built-in AI-powered notebook enables you to quickly summarize pages and pages of notes into concise bullets in a script font that can be easily shared directly from the notebook tab. You can also refine your notes into a script font, so it becomes legible while maintaining the look and feel of handwriting.
...
Read the original on www.aboutamazon.com »
To add this web app to your iOS home screen tap the share button and select "Add to the Home Screen".
10HN is also available as an iOS App
If you visit 10HN only rarely, check out the the best articles from the past week.
If you like 10HN please leave feedback and share
Visit pancik.com for more.